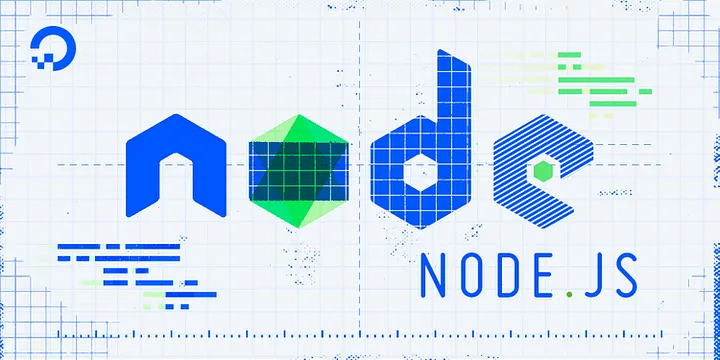
How to Set Up a Node.js Application for Production on Ubuntu 24.04
Node.js is an open-source JavaScript runtime that allows developers to build scalable server-side applications. This guide will show how to set up a production-ready Node.js environment on Ubuntu 24.04 using PM2 for process management and Nginx as a reverse proxy for secure and efficient request handling.
Step 1: Update Your System
Before installing Node.js, update all existing packages to avoid conflicts. Run the following command:
sudo apt update && sudo apt upgrade -y
Step 2: Install Node.js and npm
The best way to install the latest Long-Term Support (LTS) version of Node.js is by using the NodeSource repository.
Download and run the NodeSource setup script:
curl -fsSL https://deb.nodesource.com/setup_lts.x | sudo bash –
Now, install Node.js and npm:
sudo apt install -y nodejs
Verify that Node.js and npm are installed correctly:
node -v
npm -v
To install build tools for compiling native add-ons, run:
sudo apt install -y build-essential
Step 3: Create a Simple Node.js Application
First, create a directory for your application and navigate into it:
mkdir myapp && cd myapp
Create a new JavaScript file for the application:
nano server.js
Add the following code:
const http = require(‘http’);
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader(‘Content-Type’, ‘text/plain’);
res.end(‘Hello, World!\n’);
});
server.listen(port, () => {
console.log(Server running at http://localhost:${port}/
);
});
Save and exit the editor.
To test the application, run:
node server.js
Open another terminal and verify that it’s working:
If everything is working, press CTRL+C to stop the server.
Step 4: Install and Configure PM2
PM2 is a process manager for Node.js that allows applications to run in the background and restart automatically if they crash.
Install PM2 globally using npm:
sudo npm install -g pm2
Start the Node.js application with PM2:
pm2 start server.js
PM2 will display the running processes, showing that the app is online.
To automatically start the application on system reboot, run:
pm2 startup
PM2 will display a command that you need to execute. Copy and run that command to enable automatic startup.
To save the process list and ensure the application restarts after reboots, run:
pm2 save
Step 5: Install and Configure Nginx as a Reverse Proxy
Nginx will be used as a reverse proxy to handle incoming requests and forward them to the Node.js application.
Install Nginx using:
sudo apt install nginx -y
Start and enable Nginx:
sudo systemctl start nginx
sudo systemctl enable nginx
Create a new Nginx configuration file for the application:
sudo nano /etc/nginx/sites-available/myapp
Add the following configuration:
server {
listen 80;
server_name yourdomain.com;
location / {
proxy_pass http://localhost:3000;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection ‘upgrade’;
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Save the file and create a symbolic link to enable it:
sudo ln -s /etc/nginx/sites-available/myapp /etc/nginx/sites-enabled/
Check if the configuration is valid:
sudo nginx -t
Restart Nginx to apply the changes:
sudo systemctl restart nginx
Step 6: Secure the Application with Let’s Encrypt SSL
To enable HTTPS, install Certbot:
sudo apt install certbot python3-certbot-nginx -y
Run Certbot to obtain an SSL certificate for your domain:
sudo certbot –nginx -d yourdomain.com
Certbot will configure Nginx to use SSL automatically. To verify the renewal process, run:
sudo certbot renew –dry-run
Now, your Node.js application is running with HTTPS through Nginx.
Step 7: Test the Production Setup
Check if the application is running:
pm2 list
Ensure Nginx is active:
sudo systemctl status nginx
Visit your domain in a browser:
If everything is set up correctly, you should see Hello, World! displayed in your browser.
Conclusion
You have successfully deployed a Node.js application on Ubuntu 24.04 using PM2 and Nginx. The application runs as a background service, restarts automatically, and is secured with HTTPS.
Would you like help with database integration, scaling your application, or setting up a load balancer? Let me know! ?