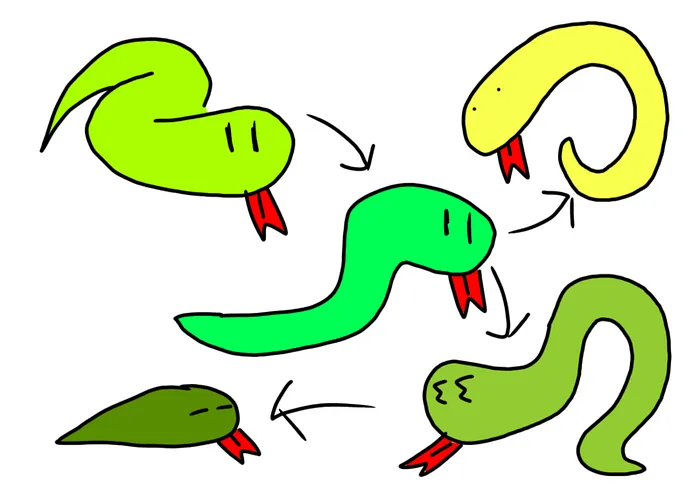
Advanced Python Features You Should Know
Python is a powerful and versatile language, but many of its most useful features are often overlooked by beginners. In this article, we’ll explore some Python techniques that will elevate your skills, including tuple unpacking, list comprehensions, magic methods, decorators, and more.
If I had known these concepts earlier in my coding journey, I could have saved a lot of time. So let’s dive in!
Tuple Unpacking and the * Operator
Tuple unpacking lets you assign multiple values to variables in a single line.
Example:
person = [‘bob’, 30, ‘male’]
name, age, gender = person
Now, name = ‘bob’, age = 30, and gender = ‘male’.
Using * to collect remaining values:
fruits = [‘apple’, ‘orange’, ‘pear’, ‘pineapple’, ‘durian’, ‘banana’]
first, second, *others = fruits
first = ‘apple’, second = ‘orange’, others = [‘pear’, ‘pineapple’, ‘durian’, ‘banana’].
List, Set, and Dictionary Comprehensions
List comprehensions allow you to create lists efficiently.
l1 = [i for i in range(1, 4)] → [1, 2, 3]
l2 = [i * 2 for i in range(1, 4)] → [2, 4, 6]
l3 = [i ** 2 for i in range(1, 4)] → [1, 4, 9]
l4 = [i for i in range(1, 4) if i % 2 == 1] → [1, 3]
Similarly, you can create sets and dictionaries.
set1 = {i for i in range(1, 4)} → {1, 2, 3}
d1 = {i: i ** 2 for i in range(1, 4)} → {1: 1, 2: 4, 3: 9}
Ternary Operator (One-Line If-Else Statements)
score = 57
grade = ‘A*’ if score > 90 else ‘pass’ if score > 50 else ‘fail’
This is equivalent to a full if-elif-else block but in a single line.
Magic Methods in Python Classes
Magic methods start with double underscores (__). They allow you to customize class behavior.
Example:
class Dog():
def init(self, name, age):
self.name = name
self.age = age
def str(self):
return f’Dog(name={self.name}, age={self.age})’
def gt(self, otherDog):
return self.age > otherDog.age
dog1 = Dog(‘rocky’, 4)
dog2 = Dog(‘fifi’, 2)
print(dog1) → Dog(name=rocky, age=4)
print(dog1 > dog2) → True (because 4 > 2)
**Using *args and kwargs
*args collects extra positional arguments as a tuple.
def test(a, b, *args):
print(f'{a=} {b=} {args=}’)
test(1, 2, 3, 4, 5) → a=1, b=2, args=(3,4,5)
**kwargs collects extra keyword arguments as a dictionary.
def test(a, b, **kwargs):
print(f'{a=} {b=} {kwargs=}’)
test(a=1, b=2, c=3, d=4) → a=1, b=2, kwargs={‘c’: 3, ‘d’: 4}
Importing from Multiple Python Files
helper.py
def test123():
print(‘test123 is called’)
main.py
from helper import test123
test123() → “test123 is called”
Learning how to organize code across multiple files is essential for real-world projects.
Using if name == ‘main’
This ensures that certain lines of code run only if the file is executed directly.
helper.py
def test123():
print(‘test123 is called’)
if name == ‘main‘:
print(‘This runs only if helper.py is executed directly’)
If imported into another file, this print statement will not execute.
Truthy & Falsy Values
Falsy values: 0, None, ”, [], {}
Truthy values: any non-empty sequence or non-zero number
Example:
if 0: print(‘Won’t print’)
if 1: print(‘Prints’)
if ‘a’: print(‘Prints’)
if {}: print(‘Won’t print’)
Break, Continue, and Pass
for i in [1,2,3,4,5]:
if i == 3:
break
print(i)
Stops at 2
for i in [1,2,3,4,5]:
if i == 3:
continue
print(i)
Skips 3 but prints 1, 2, 4, 5
Try-Except-Finally Blocks
try:
# risky code
except:
# handle error
finally:
# always executes
Handling exceptions properly prevents crashes.
Decorators
Decorators modify function behavior.
def add_exclamation_mark(your_function):
def inner(*args, **kwargs):
return your_function(*args, **kwargs) + “!”
return inner
@add_exclamation_mark
def greet(name):
return f’Hello {name}’
print(greet(‘Tim’)) → “Hello Tim!”
Generators and Yield
def simple_generator():
yield ‘apple’
yield ‘orange’
yield ‘pear’
for fruit in simple_generator():
print(fruit)
apple, orange, pear
Lambda Functions
add = lambda x, y: x + y
print(add(3, 5)) → 8
test = lambda: ‘hello’
print(test()) → ‘hello’
Multiprocessing in Python
import multiprocessing
import time
import datetime
def yourfunction(x):
start = datetime.datetime.now()
time.sleep(1)
end = datetime.datetime.now()
return f’x={x} start at {start}, end at {end}’
if name == ‘main‘:
with multiprocessing.Pool(processes=3) as pool:
data = pool.map(yourfunction, [1, 2, 3, 4, 5, 6, 7])
for row in data:
print(row)